The Angular Directive md-contact-chips, is an input control that is built on MD-chips and uses the md-auto-complete element. The contact chip component has an expression that lists the contacts. The user selects one of it and then adds it to the list of available chips.
The <md-contact-chips> is an input component based on md-chips. It is used mainly for labels.
Attributes
The table lists the parameters and descriptions of various properties of md-contact-chips.
S.No | Parameter | Description |
---|---|---|
1 | * ng-model | It is used to bind a list of items. |
2 | * md-contacts | This is expected to return from a contact matching test, $ query. |
3 | * md-contact-name | * md-contact-name is the field name of the contact object that represents the contact’s name. |
4 | * md-contact-email | The contact object’s field name represents the contact’s email address. |
5 | * md-contact-image | The contact object’s field name represents the contact’s image. |
6 | placeholder | Placeholder text is sent for input. |
7 | secondary-placeholder | If there is at least one item in the list, the placeholder text will be sent to the input displayed. |
8 | filter-selected | It is used to filter selected contacts from the list of suggestions in auto-complete. |
Example 1:
The example shows the use of the md-contact-chips instruction and the uses of angular contact chips.
app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { MatChipsModule } from '@angular/material/chips';
import { AppComponent } from './app.component';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
@NgModule({
imports:
[ BrowserModule,
FormsModule,
MatChipsModule,
BrowserAnimationsModule],
declarations: [ AppComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
app.component.html
<p>Default Chip List</p>
<mat-chip-list aria-label="Fish selection">
<mat-chip color="primary" selected>
Primary theme chip
</mat-chip>
<mat-chip color="accent" selected>
Accent theme chip
</mat-chip>
<mat-chip color="warn" selected>
Warn theme chip
</mat-chip>
<mat-chip>Default theme chip </mat-chip>
</mat-chip-list>
<br><br><br>
<p>Stacked Chip List</p>
<mat-chip-list class="mat-chip-list-stacked"
aria-label="Fish selection">
<mat-chip color="primary" selected>
Primary theme chip
</mat-chip>
<mat-chip color="accent" selected>
Accent theme chip
</mat-chip>
<mat-chip color="warn" selected>
Warn theme chip
</mat-chip>
<mat-chip>Default theme chip </mat-chip>
</mat-chip-list>
Output:
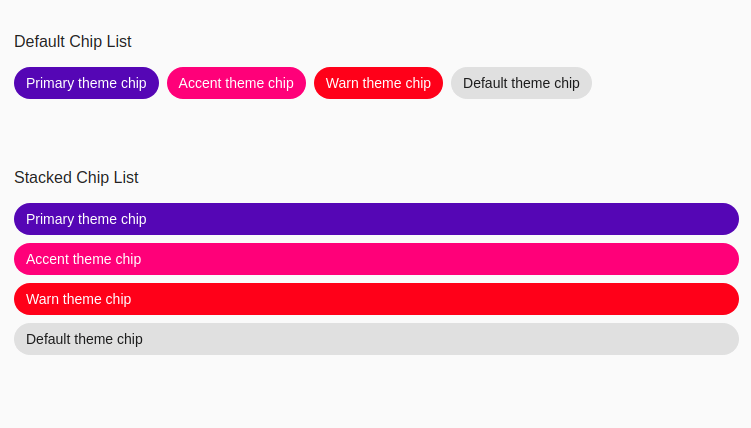
Example 2:
app.module.html
<div ng-controller="ContactChipDemoCtrl as ctrl" layout="column" ng-cloak="" class="chipsdemoContactChips" ng-app="MyApp">
<md-content class="md-padding autocomplete" layout="column">
<md-contact-chips ng-model="ctrl.contacts" md-contacts="ctrl.querySearch($query)" md-contact-name="name" md-contact-image="image" md-contact-email="email" md-require-match="true" md-highlight-flags="i" filter-selected="ctrl.filterSelected" placeholder="To">
</md-contact-chips>
<md-list class="fixedRows">
<md-subheader class="md-no-sticky">Contacts</md-subheader>
<md-list-item class="md-2-line contact-item" ng-repeat="(index, contact) in ctrl.allContacts" ng-if="ctrl.contacts.indexOf(contact) < 0">
<img ng-src="{{contact.image}}" class="md-avatar" alt="{{contact.name}}">
<div class="md-list-item-text compact">
<h3>{{contact.name}}</h3>
<p>{{contact.email}}</p>
</div>
</md-list-item>
<md-list-item class="md-2-line contact-item selected" ng-repeat="(index, contact) in ctrl.contacts">
<img ng-src="{{contact.image}}" class="md-avatar" alt="{{contact.name}}">
<div class="md-list-item-text compact">
<h3>{{contact.name}}</h3>
<p>{{contact.email}}</p>
</div>
</md-list-item>
</md-list>
</md-content>
</div>
app.component.css
.chipsdemoContactChips md-content.autocomplete {
min-height: 250px; }
.chipsdemoContactChips .md-item-text.compact {
padding-top: 8px;
padding-bottom: 8px; }
.chipsdemoContactChips .contact-item {
box-sizing: border-box; }
.chipsdemoContactChips .contact-item.selected {
opacity: 0.5; }
.chipsdemoContactChips .contact-item.selected h3 {
opacity: 0.5; }
.chipsdemoContactChips .contact-item .md-list-item-text {
padding: 14px 0; }
.chipsdemoContactChips .contact-item .md-list-item-text h3 {
margin: 0 !important;
padding: 0;
line-height: 1.2em !important; }
.chipsdemoContactChips .contact-item .md-list-item-text h3, .chipsdemoContactChips .contact-item .md-list-item-text p {
text-overflow: ellipsis;
white-space: nowrap;
overflow: hidden; }
@media (min-width: 900px) {
.chipsdemoContactChips .contact-item {
float: left;
width: 33%; } }
.chipsdemoContactChips md-contact-chips {
margin-bottom: 10px; }
.chipsdemoContactChips .md-chips {
padding: 5px 0 8px; }
.chipsdemoContactChips .fixedRows {
height: 250px;
overflow: hidden; }
app.component.ts
(function () {
'use strict';
angular
.module('MyApp')
.controller('ContactChipDemoCtrl', DemoCtrl);
function DemoCtrl ($timeout, $q) {
var self = this;
self.querySearch = querySearch;
self.allContacts = loadContacts();
self.contacts = [self.allContacts[0]];
self.filterSelected = true;
/**
* Search for contacts.
*/
function querySearch (query) {
var results = query ?
self.allContacts.filter(createFilterFor(query)) : [];
return results;
}
/**
* Create filter function for a query string
*/
function createFilterFor(query) {
var lowercaseQuery = angular.lowercase(query);
return function filterFn(contact) {
return (contact._lowername.indexOf(lowercaseQuery) != -1);;
};
}
function loadContacts() {
var contacts = [
'Marina Augustine',
'Oddr Sarno',
'Nick Giannopoulos',
'Narayana Garner',
'Anita Gros',
'Megan Smith',
'Tsvetko Metzger',
'Hector Simek',
'Some-guy withalongalastaname'
];
return contacts.map(function (c, index) {
var cParts = c.split(' ');
var contact = {
name: c,
email: cParts[0][0].toLowerCase() + '.' + cParts[1].toLowerCase() + '@example.com',
image: ' ' + index
};
contact._lowername = contact.name.toLowerCase();
return contact;
});
}
}
})();
Output:
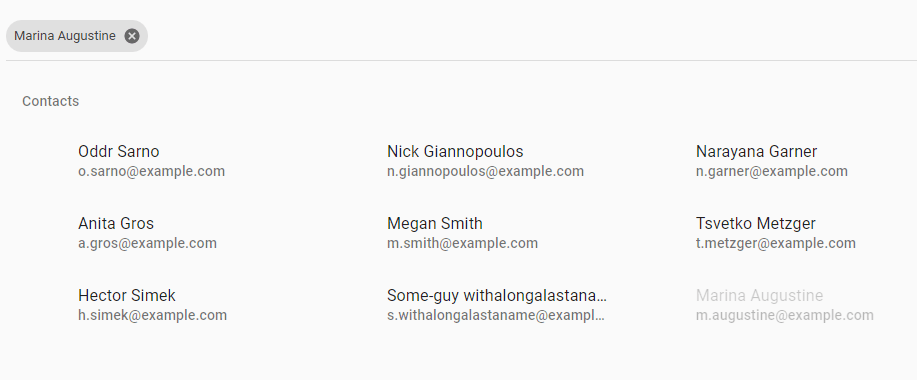
Leave a Reply